1. Yad Examples
It is posible to create quite advanced programs with YAD.
Here are some example usage of YAD.
1.1. Simple run dialog with history
This is a simple run dialog with history.
The dialog has 3 buttons, Clear History
, Cancel
and Execute
Clear History
Clears the history
Cancel
Close the run dialog
Execute
Runs the command
This script don’t have any error check to se if the command is successful or not.
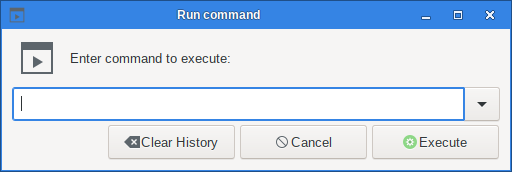
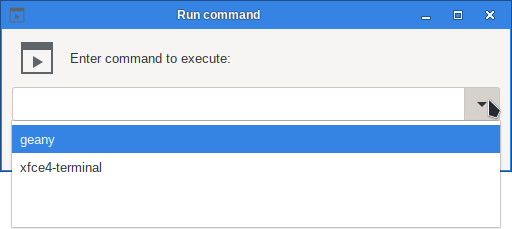
#!/bin/bash
XTERM="xterm"
export SCRIPT=$(readlink -f $0)
export PWD=$(dirname $SCRIPT)
# Create history file
mkdir -p ${XDG_CACHE_HOME:-$HOME/.cache}/
export HISTFILE=${XDG_CACHE_HOME:-$HOME/.cache}/ix-run.history
# Create history file
if [ ! -f $HISTFILE ]; then
mkdir -p ${XDG_CACHE_HOME:-$HOME/.cache}/
echo "\n" > $HISTFILE
fi
# Delete history file
Clear_History(){
rm $HISTFILE
yad --fixed \
--title="History" \
--window-icon="applications-system" \
--text="\n History Cleared \n" \
--timeout="1" \
--no-buttons
echo "\n" > $HISTFILE
kill $YAD_PID
exec $SCRIPT
}
export -f Clear_History
# Create and run dialog
TITLE="Run command"
TEXT="\nEnter command to execute:\n"
rcmd=$(yad --entry \
--width=500 \
--center \
--window-icon="gtk-execute" \
--name="${0##/}" \
--title="$TITLE" \
--text="$TEXT" \
--image="gtk-execute" \
--editable \
--rest $HISTFILE \
--button="Clear History!gtk-clear:bash -c Clear_History" \
--button="yad-cancel:1" \
--button="yad-execute:0")
[ -z "$rcmd" ] && exit 0
# Run command
case $rcmd in
http://*|https://*|ftp://*)
xdg-open $rcmd &
;;
mailto://*)
xdg-email $rcmd &
;;
man://*)
eval $XTERM -e "man ${rcmd#man://}" &
;;
telnet*|ssh*)
eval $XTERM -e "$rcmd" &
;;
*)
eval $rcmd &
;;
esac
# Add command to history
grep -q -F "$rcmd" $HISTFILE || sed -i "1 i $rcmd" $HISTFILE
exit 0
1.2. Frontend for find
This is a example based on Victor Ananjevsky’s Frontend for find(1), but i could not get it to work on Salix 15.0 properly.
The problem was that when i tried to open a result it dident open, the error was:
xdg-open: unexpected argument '6'
Try 'xdg-open --help' for more information.
So i had to make a extra function to open files
function xopen {
xdg-open "$1" &> /dev/null
}
export -f xopen
and change the following part --dclick-action="xdg-open '%s'"
to --dclick-action='bash -c "xopen %s"'
,
now it will open the file you doubble click on.
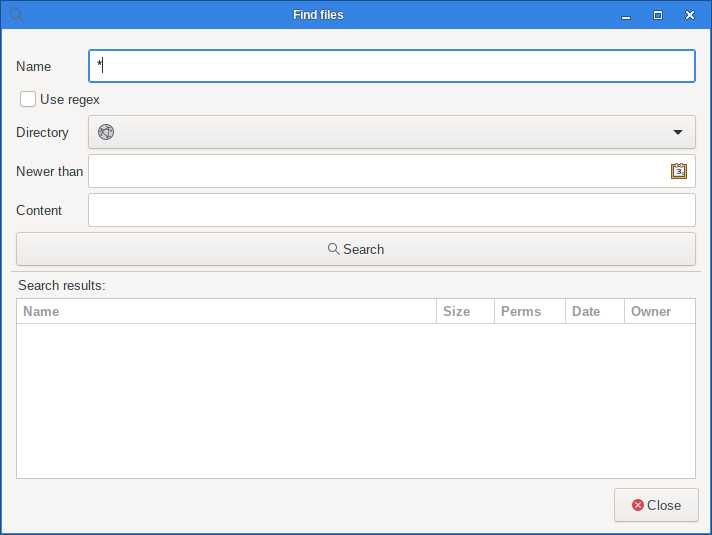
#!/bin/bash
export find_cmd='@sh -c "run_find %1 %2 %3 %4 %5"'
export fpipe=$(mktemp -u --tmpdir find.XXXXXXXX)
mkfifo "$fpipe"
trap "rm -f $fpipe" EXIT
KEY=$RANDOM
function run_find {
echo "6:@disabled@"
if [[ $2 != TRUE ]]; then
ARGS="-name '$1'"
else
ARGS="-regex '$1'"
fi
if [[ -n "$4" ]]; then
dt=$(echo "$4" | awk -F. '{printf "%s-%s-%s", $3, $2, $1}')
d1=$(date +%j -d $dt)
d2=$(date +%j)
d=$(($d1 - $d2))
ARGS+=" -ctime $d"
fi
if [[ -n "$5" ]]; then
ARGS+=" -exec grep -q -E '$5' {} \;"
fi
ARGS+=" -printf '%p\n%s\n%M\n%TD %TH:%TM\n%u/%g\n'"
echo -e '\f' >> "$fpipe"
eval find "$3" $ARGS >> "$fpipe"
echo "6:$find_cmd"
}
export -f run_find
function xopen {
xdg-open "$1" &> /dev/null
}
export -f xopen
exec 3<> $fpipe
yad --plug=$KEY --tabnum=1 --form --date-format="%Y-%m-%d" \
--field=$"Name" '*' --field=$"Use regex:chk" 'no' \
--field=$"Directory:dir" '' --field=$"Newer than:dt" '' \
--field=$"Content" '' --field="yad-search:fbtn" "$find_cmd" &
yad --plug=$KEY --tabnum=2 --list --no-markup --dclick-action='bash -c "xopen %s"' \
--text $"Search results:" --column=$"Name" --column=$"Size:sz" --column=$"Perms" \
--column=$"Date" --column=$"Owner" --search-column=1 --expand-column=1 <&3 &
yad --paned --key=$KEY --button="yad-close:1" --width=700 --height=500 \
--title=$"Find files" --window-icon="find"
exec 3>&-
1.3. System informations
This example is based on Victor Ananjevsky’s System informations
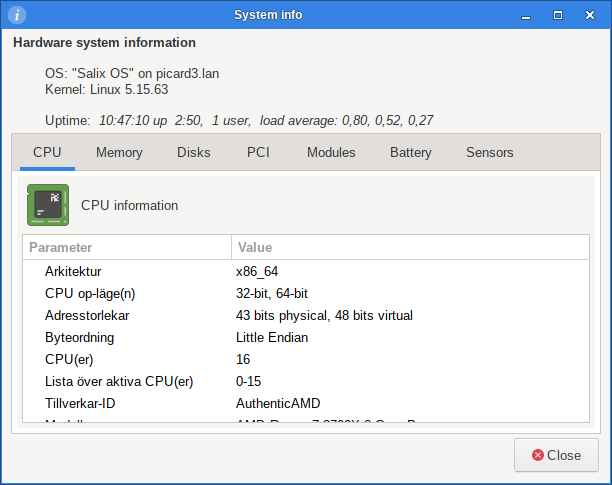
#!/bin/bash
KEY=$RANDOM
function show_mod_info {
TXT="\\n<span face='Monospace'>$(modinfo $1 | sed 's/&/\&/g;s/</\</g;s/>/\>/g')</span>"
yad --title="Module information" \
--window-icon="application-x-addon" \
--button="yad-close" \
--width=500 \
--image="application-x-addon" --text="$TXT"
}
export -f show_mod_info
# CPU tab
lscpu | sed -r "s/:[ ]*/\n/" |\
yad --plug=$KEY --tabnum=1 --image=cpu --text="CPU information" \
--list --no-selection --column="Parameter" --column="Value" &
# Memory tab
sed -r "s/:[ ]*/\n/" /proc/meminfo |\
yad --plug=$KEY --tabnum=2 --image=media-memory \
--text="Memory usage information" \
--list --no-selection --column="Parameter" --column="Value" &
# Harddrive tab
df -T | tail -n +2 | awk '{printf "%s\n%s\n%s\n%s\n%s\n%s\n", $1,$7, $2, $3, $4, $6}' |\
yad --plug=$KEY --tabnum=3 --image=drive-harddisk \
--text="Disk space usage" \
--list --no-selection --column="Device" \
--column="Mountpoint" --column="Type" \
--column="Total:sz" --column="Free:sz" \
--column="Usage:bar" &
# PCI tab
lspci -vmm | sed 's/\&/\&/g' | grep -E "^(Slot|Class|Vendor|Device|Rev):" | cut -f2 |\
yad --plug=$KEY --tabnum=4 --text="PCI bus devices" \
--list --no-selection --column="ID" --column="Class" \
--column="Vendor" --column="Device" --column="Rev" &
# Modules tab
awk '{printf "%s\n%s\n%s\n", $1, $3, $4}' /proc/modules | sed "s/[,-]$//" |\
yad --plug=$KEY --tabnum=5 --text="Loaded kernel modules" \
--image="application-x-addon" --image-on-top \
--list --dclick-action='bash -c "show_mod_info %s"' \
--column="Name" --column="Used" --column="Depends" &
# Battery tab
( acpi -i ; acpi -a ) | sed -r "s/:[ ]*/\n/" | yad --plug=$KEY --tabnum=6 \
--image=battery --text="Battery state" --list --no-selection \
--column="Device" --column="Details" &
# Sensors tab
SENSORS=($(sensors | grep -E '^[^:]+$'))
sid=1
cid=1
for s in ${SENSORS[@]}; do
echo -e "s$sid\n<b>$s</b>\n"
sensors -A "$s" | tail -n +2 | while read ln; do
[[ $ln == "" ]] && continue
echo "$cid:s$sid"
echo $ln | sed -r 's/:[ ]+/\n/'
((cid++))
done
((sid++))
done | yad --plug=$KEY --tabnum=7 --text="Temperature sensors information" \
--list --tree --tree-expanded --no-selection --column="Sensor" --column="Value" &
# Main dialog
TXT="<b>Hardware system information</b>\\n\\n"
TXT+="\\tOS: $(lsb_release -ds) on $(hostname)\\n"
TXT+="\\tKernel: $(uname -sr)\\n\\n"
TXT+="\\tUptime: <i>$(uptime)</i>"
yad --notebook --window-icon="dialog-information" \
--width=600 --height=450 --title="System info" --text="$TXT" --button=yad-close \
--key=$KEY --tab="CPU" --tab="Memory" --tab="Disks" --tab="PCI" --tab="Modules" \
--tab="Battery" --tab="Sensors" --active-tab=${1:-1}
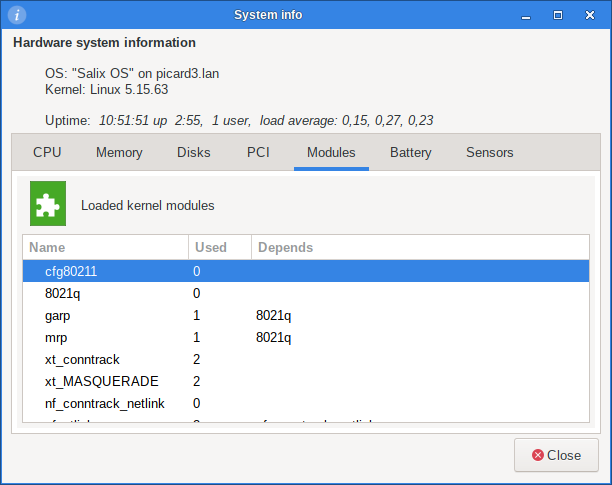
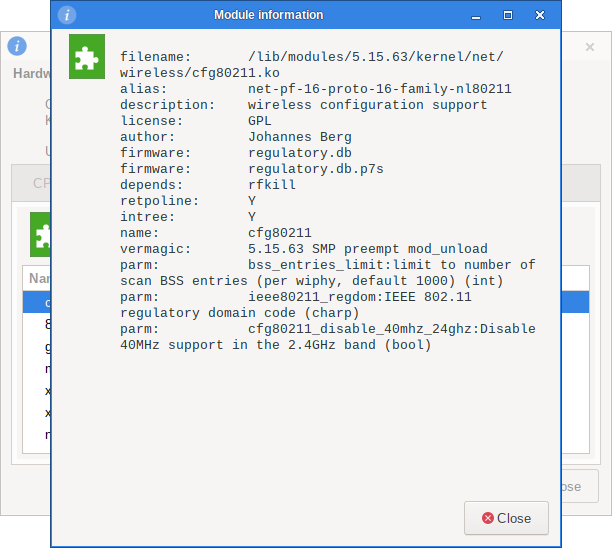
1.4. Yes/No dialog
This example shows how to get a dialog with Yes/No buttons and how to use the users responce.
Display a dialog, asking Microsoft Windows has been found! Would you like to remove it?.
The return code will be 0 (true in shell) if YES is selected, and 1 (false) if NO is selected.
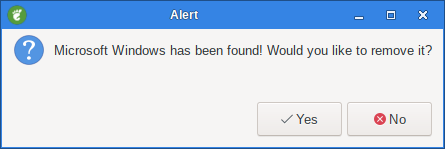
#!/bin/bash
yad --image="dialog-question" \
--title "Alert" \
--text "Microsoft Windows has been found! Would you like to remove it?" \
--button="yad-yes:0" \
--button="yad-no:1" \
ret=$?
[[ $ret -eq 1 ]] && echo "Ok, i will not remove Windows"
[[ $ret -eq 0 ]] && echo "Great! I will remove Windows now..."
1.5. Notification icon
Show an icon in the notification area.
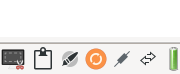
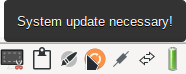
#!/bin/bash
yad --notification \
--image=update \
--text "System update necessary!" \
--command "xterm -e apt-get upgrade"
1.6. iDesk Desktop Icon Creator/Editor
This example is a program to create/edit a iDesk icon.
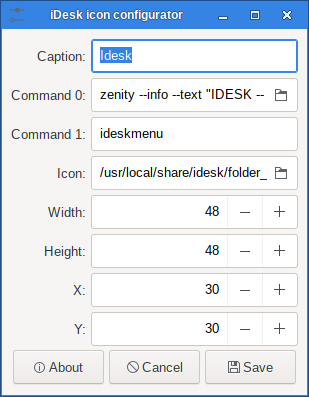
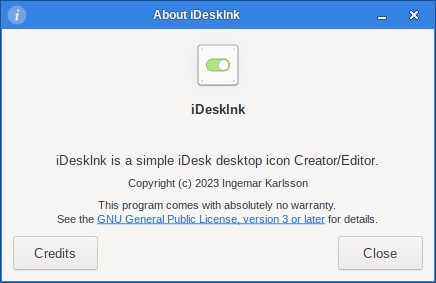
#!/bin/bash
########################################################################
#
# AUTHOR: (c) Ingemar Karlsson <ingemar@ingk.se>
# WWW: ingk.se
# LICENSE: GPL3 (https://www.gnu.org/licenses/gpl-3.0.html)
# REQUIRES: bash, idesk, yad
# NAME: idesklnk
# DESCRIPTION: iDesklnk cretates/edit idesk desktop icons.
# VERSION: 1.0.0 (2023)
#
########################################################################
########################################################################
# Set configuration directory
# Depending of witch version of iDesk you are running the directory for
# iDesk desktop icons files has different directory path.
CONF_DIR="$HOME/.config/idesktop"
#CONF_DIR="$HOME/.idesktop"
########################################################################
# Set the program version
PVERSION="1.0.0"
########################################################################
# Default values
export F_NAME=0
export CAPTION=""
export COMMAND0=""
export COMMAND1="ideskmenu"
export ICON="/usr/share/icons"
export ICON_SIZE=0
export WIDTH=48
export HEIGHT=48
export X=0
export Y=0
export BUTTON2_TXT="Create!gtk-apply"
export IDESK_LNK_FILE=""
export RESULT=""
########################################################################
# Clean upfunction
function clean_up() {
unset CAPTION
unset COMMAND0
unset COMMAND1
unset ICON
unset ICON_SIZE
unset WIDTH
unset HEIGHT
unset X
unset Y
unset IDESK_LNK_FILE
}
export -f clean_up
########################################################################
# Save file function
function save_link() {
IFS='|'
arrRES=(${RESULT})
CAPTION=${arrRES[0]}
COMMAND0=${arrRES[1]}
COMMAND1=${arrRES[2]}
ICON=${arrRES[3]}
WIDTH=${arrRES[4]}
HEIGHT=${arrRES[5]}
X=${arrRES[6]}
Y=${arrRES[7]}
unset IFS
if [[ $F_NAME == 1 ]]; then
LNK_FILE="${IDESK_LNK_FILE}"
else
LNK_FILE="${COMMAND1} ${CONF_DIR}/${CAPTION// /_}.lnk"
fi
echo -e "table Icon
Caption: ${CAPTION}
Command[0]: ${COMMAND0}
Command[1]: ${LNK_FILE}
Icon: ${ICON}
Width: ${WIDTH}
Height: ${HEIGHT}
X: ${X}
Y: ${Y}
end
" > ${LNK_FILE}
# Clean up variables
clean_up
}
export -f save_link
########################################################################
# Read file function
function read_file() {
CAPTION="$(grep ^..Caption: ${IDESK_LNK_FILE} | awk -F': ' '{print $2}')"
COMMAND0="$(grep ^..Command.0.: ${IDESK_LNK_FILE} | awk -F': ' '{print $2}')"
if [[ "${COMMAND0}" == "" ]]; then
COMMAND0="$(grep ^..Command: ${IDESK_LNK_FILE} | awk -F': ' '{print $2}')"
fi
COMMAND2="$(grep ^..Command.1.: ${IDESK_LNK_FILE} | awk -F': ' '{print $2}')"
if [[ ! "$COMMAND2" == "" ]]; then
COMMAND1=$COMMAND2
fi
ICON="$(grep ^..Icon: ${IDESK_LNK_FILE} | awk -F': ' '{print $2}')"
WIDTH="$(grep ^..Width: ${IDESK_LNK_FILE} | awk -F': ' '{print $2}')"
HEIGHT="$(grep ^..Height: ${IDESK_LNK_FILE} | awk -F': ' '{print $2}')"
X="$(grep ^..X: ${IDESK_LNK_FILE} | awk -F': ' '{print $2}')"
Y="$(grep ^..Y: ${IDESK_LNK_FILE} | awk -F': ' '{print $2}')"
}
export -f read_file
########################################################################
# about dialog
function about_dlg() {
yad --about \
--window-icon=gtk-about \
--image=gtk-preferences \
--authors="Ingemar Karlsson" \
--license="GPL3" \
--comments="iDesklnk is a simple iDesk desktop icon Creator/Editor." \
--copyright="Copyright (c) 2023 Ingemar Karlsson" \
--pversion="$PVERSION" \
--pname="iDesklnk" \
--button="Close!gtk-close":1
}
export -f about_dlg
if [[ ! $1 == "" ]]; then
IDESK_LNK_FILE=$1
F_NAME=1
BUTTON2_TXT="Save!gtk-save"
read_file
fi
########################################################################
# Creat the application
RESULT=$(yad --form \
--window-icon=gtk-preferences \
--align=right \
--width=150 \
--mouse \
--title="iDesk icon configurator" \
--field="Caption:" "$CAPTION" \
--field="Command 0::SFL" "$COMMAND0" \
--field="Command 1:" "$COMMAND1" \
--field="Icon::SFL" "$ICON" \
--field="Width::NUM" "$WIDTH"!16..128!16 \
--field="Height::NUM" "$HEIGHT"!16..128!16 \
--field="X::NUM" "$X" \
--field="Y::NUM" "$Y" \
--button="About!gtk-about":"bash -c about_dlg" \
--button="Cancel!gtk-cancel":1 \
--button="$BUTTON2_TXT":0)
ret=$?
[[ $ret -eq 1 ]] && clean_up
[[ $ret -eq 0 ]] && save_link