1. Yad Notebook
Notebook is a complex dialog which swallow other dialogs in his tabs. Dialogs identifies by unique key (integer) and must be runs in a special plug mode (--plug option). Following example runs notebook dialog with two tabs, first has a simple text and second is an entry dialog.
#!/bin/bash
yad --plug=12345 --tabnum=1 --text="first tab with text" &> res1 & \
yad --plug=12345 --tabnum=2 --text="second tab" --entry &> res2 & \
yad --notebook --key=12345 --tab="Tab 1" --tab="Tab 2"
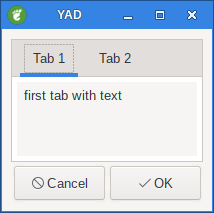
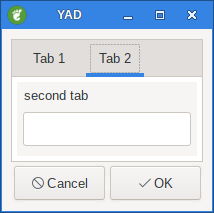
1.1. --tab=TEXT
Add tab with specified label to notebook.
TEXT
may be in a form LABEL[!ICON[!TOOLTIP]]
where !
is an item separator.
For stack mode TEXT
for label uses as is.
1.2. --tab-pos=TYPE
Set the tabs position.
Value may be top
, bottom
, left
, or right
.
Default is top
.
For stack mode only top or bottom positions available.
1.2.1. --tab-pos=top
#!/bin/bash yad --plug=12345 --tabnum=1 --text="first tab with text" &> res1 & \ yad --plug=12345 --tabnum=2 --text="second tab" --entry &> res2 & \ yad --notebook --tab-pos=top --key=12345 --tab="Tab 1" --tab="Tab 2"
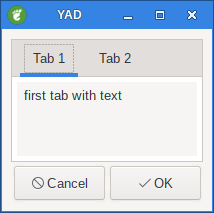
1.2.2. --tab-pos=left
#!/bin/bash yad --plug=12345 --tabnum=1 --text="first tab with text" &> res1 & \ yad --plug=12345 --tabnum=2 --text="second tab" --entry &> res2 & \ yad --tab-pos=left --notebook --key=12345 --tab="Tab 1" --tab="Tab 2"
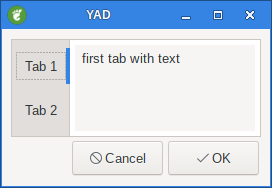
1.2.3. --tab-pos=right
#!/bin/bash
yad --plug=12345 --tabnum=1 --text="first tab with text" &> res1 & \
yad --plug=12345 --tabnum=2 --text="second tab" --entry &> res2 & \
yad --notebook--tab-pos=right --key=12345 --tab="Tab 1" --tab="Tab 2"
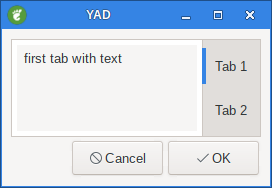
1.2.4. --tab-pos=bottom
#!/bin/bash
yad --plug=12345 --tabnum=1 --text="first tab with text" &> res1 & \
yad --plug=12345 --tabnum=2 --text="second tab" --entry &> res2 & \
yad --notebook--tab-pos=bottom --key=12345 --tab="Tab 1" --tab="Tab 2"
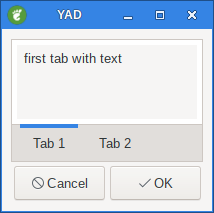
1.3. --tab-borders=NUMBER
Set the borders width around widget in tabs.
#!/bin/bash
yad --plug=12345 --tabnum=1 --text="first tab with text" &> res1 & \
yad --plug=12345 --tabnum=2 --text="second tab" --entry &> res2 & \
yad --notebook --tab-borders=20 --key=12345 --tab="Tab 1" --tab="Tab 2"
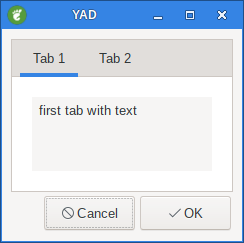
1.4. --active-tab=NUMBER
Set active tab.
#!/bin/bash
yad --plug=12345 --tabnum=1 --text="first tab with text" &> res1 & \
yad --plug=12345 --tabnum=2 --text="second tab" --entry &> res2 & \
yad --notebook --active-tab=2 --key=12345 --tab="Tab 1" --tab="Tab 2"
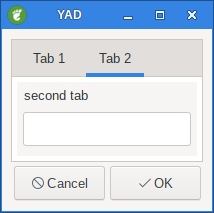
2. --expand
Expand all tabs to full width of a dialog window.
#!/bin/bash
yad --plug=12345 --tabnum=1 --text="first tab with text" &> res1 & \
yad --plug=12345 --tabnum=2 --text="second tab" --entry &> res2 & \
yad --expand --notebook --key=12345 --tab="Tab 1" --tab="Tab 2" --width=400
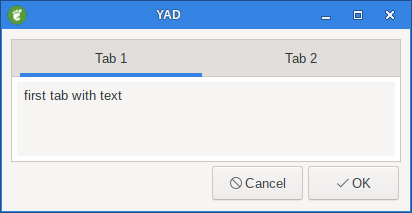
3. --stack
Use stack mode (GtkStack instead of GtkNotebook).
#!/bin/bash
yad --plug=12345 --tabnum=1 --text="first tab with text" &> res1 & \
yad --plug=12345 --tabnum=2 --text="second tab" --entry &> res2 & \
yad --stack --notebook --key=12345 --tab="Tab 1" --tab="Tab 2" --width=400
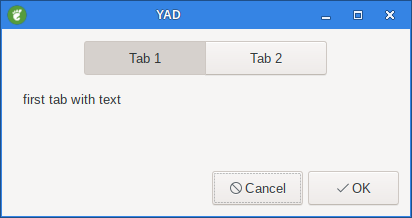
4. Examples
4.1. gtksettings
This demostrate the usage of notebook dialog. Three tabs with different settings of gtk+-2.0
I had to change a few things for it to work on Salix OS.
#! /bin/sh
# -*- mode: sh -*-
#
# Author: Victor Ananjevsky <ananasik@gmail.com>, 2013
#
KEY="12345"
res1=$(mktemp /tmp/iface1.XXXXXXXX)
res2=$(mktemp /tmp/iface2.XXXXXXXX)
res3=$(mktemp /tmp/iface3.XXXXXXXX)
rc_file="${XDG_CONFIG_HOME:-$HOME/.config}/gtk-2.0/gtkrc"
rc_file="$HOME/.gtkrc-2.0"
# parse rc file
PARSER='
BEGIN { FS="="; OFS="\n"; }
/^gtk-theme-name/ {printf "GTKTHEME=%s\n", $2}
/^gtk-key-theme-name/ {printf "KEYTHEME=%s\n", $2}
/^gtk-icon-theme-name/ {printf "ICONTHEME=%s\n", $2}
/^gtk-cursor-theme-name/ {printf "CURSORTHEME=%s\n", $2}
/^gtk-toolbar-style/ {printf "TBSTYLE=%s\n", $2}
/^gtk-toolbar-icon-size/ {printf "TBICONSIZE=%s\n", $2}
/^gtk-button-images/ {printf "BUTTONIMAGE=%s\n", ($2 == 1) ? "TRUE" : "FALSE"}
/^gtk-menu-images/ {printf "MENUIMAGE=%s\n", ($2 == 1) ? "TRUE" : "FALSE"}
/^gtk-font-name/ {printf "FONTDESCR=%s\n", $2}
/^gtk-xft-antialiasing/ {printf "ANTIALIASING=%s\n", ($2 == 1) ? "TRUE" : "FALSE"}
/^gtk-xft-hinting/ {printf "HINTING=%s\n", ($2 == 1) ? "TRUE" : "FALSE"}
/^gtk-xft-hintstyle/ {printf "HINTSTYLE=%s\n", $2}
/^gtk-xft-rgba/ {printf "RGBA=%s\n", $2}
/^gtk-color-scheme/ {printf "COLORSCHEME=%s\n", $2}
'
eval $(sed -r "s/[ \t]*=[ \t]*/=/" $rc_file | awk "$PARSER")
# create list of gtk themes
themelist=
keythemelist="Default"
for d in /usr/share/themes/*; do
theme=${d##*/}
if [[ -e $d/gtk-2.0/gtkrc ]]; then
[[ $themelist ]] && themelist="$themelist!"
[[ $theme == $GTKTHEME ]] && theme="^$theme"
themelist+="$theme"
fi
if [[ -e $d/gtk-2.0-key/gtkrc ]]; then
[[ $theme == $KEYTHEME ]] && theme="^$theme"
keythemelist+="!$theme"
fi
done
# create list of icon and cursor themes
iconthemelist="Default"
cursorthemelist="Default"
for d in /usr/share/icons/*; do
theme=${d##*/}
if [[ -e $d/index.theme ]]; then
[[ $theme == $ICONTHEME ]] && theme="^$theme"
iconthemelist+="!$theme"
fi
if [[ -d $d/cursors ]]; then
[[ $theme == $CURSORTHEME ]] && theme="^$theme"
cursorthemelist+="!$theme"
fi
done
# create toolbar styles list
tbstylelist="$(eval_gettext 'Icons only')"!
[[ $TBSTYLE == GTK_TOOLBAR_TEXT ]] && tbstylelist+="^"
tbstylelist+="$(eval_gettext 'Text only')"!
[[ $TBSTYLE == GTK_TOOLBAR_BOTH ]] && tbstylelist+="^"
tbstylelist+="$(eval_gettext 'Text below icons')"!
[[ $TBSTYLE == GTK_TOOLBAR_BOTH_HORIZ ]] && tbstylelist+="^"
tbstylelist+="$(eval_gettext 'Text beside icons')"
# create list of toolbar icons sizes
tbiconsizelist="$(eval_gettext 'Menu')"!
[[ $TBSTYLE == GTK_ICON_SIZE_SMALL_TOOLBAR ]] && tbiconsizelist+="^"
tbiconsizelist+="$(eval_gettext 'Small toolbar')"!
[[ $TBSTYLE == GTK_ICON_SIZE_LARGE_TOOLBAR ]] && tbiconsizelist+="^"
tbiconsizelist+="$(eval_gettext 'Large toolbar')"!
[[ $TBSTYLE == GTK_ICON_SIZE_BUTTON ]] && tbiconsizelist+="^"
tbiconsizelist+="$(eval_gettext 'Buttons')"!
[[ $TBSTYLE == GTK_ICON_SIZE_DND ]] && tbiconsizelist+="^"
tbiconsizelist+="$(eval_gettext 'DND')"!
[[ $TBSTYLE == GTK_ICON_SIZE_DIALOG ]] && tbiconsizelist+="^"
tbiconsizelist+="$(eval_gettext 'Dialog')"
# theme page
yad --plug=$KEY --tabnum=1 --form --separator='\n' --quoted-output \
--field="$(eval_gettext 'GTK+ theme')::cb" "$themelist" \
--field="$(eval_gettext 'GTK+ key theme')::cb" "$keythemelist" \
--field="$(eval_gettext 'Icons theme')::cb" "$iconthemelist" \
--field="$(eval_gettext 'Cursor theme')::cb" "$cursorthemelist" \
--field="$(eval_gettext 'Toolbar style')::cb" "$tbstylelist" \
--field="$(eval_gettext 'Toolbar icon size')::cb" "$tbiconsizelist" \
--field="$(eval_gettext 'Button images'):chk" "$BUTTONIMAGE" \
--field="$(eval_gettext 'Menu images'):chk" "$MENUIMAGE" > $res1 &
# create list of hinting styles
hintstylelist="None"!
[[ $HINTSTYLE == hintslight ]] && hintstylelist+="^"
hintstylelist+="Slight"!
[[ $HINTSTYLE == hintmedium ]] && hintstylelist+="^"
hintstylelist+="Medium"!
[[ $HINTSTYLE == hintfull ]] && hintstylelist+="^"
hintstylelist+="Full"
# create list of rgba types
rgbalist="None"!
[[ $RGBA == rgb ]] && rgbalist+="^"
rgbalist+="RGB"!
[[ $RGBA == gbr ]] && rgbalist+="^"
rgbalist+="BGR"!
[[ $RGBA == vrgb ]] && rgbalist+="^"
rgbalist+="VRGB"!
[[ $RGBA == vgbr ]] && rgbalist+="^"
rgbalist+="VBGR"
# fonts page
yad --plug=$KEY --tabnum=2 --form --separator='\n' --quoted-output \
--field="Font::fn" "$FONTDESCR" --field=":lbl" "" \
--field="Use antialiasing:chk" "$ANTIALIASING" \
--field="Use hinting:chk" "$HINTING" \
--field="Hinting style::cb" "$hintstylelist" \
--field="RGBA type::cb" "$rgbalist" > $res2 &
# parse color scheme
eval $(echo -e $COLORSCHEME | tr ';' '\n' | sed -r 's/:(.*)$/="\1"/')
# colors page
yad --plug=$KEY --tabnum=3 --form --separator='\n' --quoted-output \
--field="Foreground::clr" $fg_color \
--field="Background::clr" $bg_color \
--field="Text::clr" $text_color \
--field="Base::clr" $base_color \
--field="Selected fore::clr" $selected_fg_color \
--field="Selected back::clr" $selected_bg_color \
--field="Tooltip fore::clr" $tooltip_fg_color \
--field="Tooltip back::clr" $tooltip_bg_color > $res3 &
# run main dialog
yad --window-icon=gnome-settings-theme \
--notebook --key=$KEY --tab="Theme" --tab="Fonts" --tab="Colors" \
--title="Interface settings" --image=gnome-settings-theme \
--width=400 --image-on-top --text="Common interface settings"
# recreate rc file
if [[ $? -eq 0 ]]; then
eval TAB1=($(< $res1))
eval TAB2=($(< $res2))
eval TAB3=($(< $res3))
echo -e "\n# This file was generated automatically\n" > $rc_file
echo "gtk-theme-name = \"${TAB1[0]}\"" >> $rc_file
[[ ${TAB1[1]} != Default ]] && echo "gtk-key-theme-name = \"${TAB1[1]}\"" >> $rc_file
[[ ${TAB1[2]} != Default ]] && echo "gtk-icon-theme-name = \"${TAB1[2]}\"" >> $rc_file
[[ ${TAB1[3]} != Default ]] && echo "gtk-cursor-theme-name = \"${TAB1[3]}\"" >> $rc_file
echo >> $rc_file
case ${TAB1[4]} in
"Icons only") echo "gtk-toolbar-style = GTK_TOOLBAR_ICONS" >> $rc_file ;;
"Text only") echo "gtk-toolbar-style = GTK_TOOLBAR_TEXT" >> $rc_file ;;
"Text below icons") echo "gtk-toolbar-style = GTK_TOOLBAR_BOTH" >> $rc_file ;;
"Text beside icons") echo "gtk-toolbar-style = GTK_TOOLBAR_BOTH_HORIZ" >> $rc_file ;;
esac
case ${TAB1[5]} in
"Menu") echo "gtk-toolbar-icon-size = GTK_ICON_SIZE_MENU" >> $rc_file ;;
"Small toolbar") echo "gtk-toolbar-icon-size = GTK_ICON_SIZE_SMALL_TOOLBAR" >> $rc_file ;;
"Large toolbar") echo "gtk-toolbar-icon-size = GTK_ICON_SIZE_LARGE_TOOLBAR" >> $rc_file ;;
"Buttons") echo "gtk-toolbar-icon-size = GTK_ICON_SIZE_BUTTON" >> $rc_file ;;
"DND") echo "gtk-toolbar-icon-size = GTK_ICON_SIZE_DND" >> $rc_file ;;
"Dialog") echo "gtk-toolbar-icon-size = GTK_ICON_SIZE_DIALOG" >> $rc_file ;;
esac
echo >> $rc_file
echo -n "gtk-button-images = " >> $rc_file
[[ ${TAB1[6]} == TRUE ]] && echo 1 >> $rc_file || echo 0 >> $rc_file
echo -n "gtk-menu-images = " >> $rc_file
[[ ${TAB1[7]} == TRUE ]] && echo 1 >> $rc_file || echo 0 >> $rc_file
echo >> $rc_file
echo -e "gtk-font-name = \"${TAB2[0]}\"\n" >> $rc_file
echo -n "gtk-xft-antialiasing = " >> $rc_file
[[ ${TAB2[2]} == TRUE ]] && echo 1 >> $rc_file || echo 0 >> $rc_file
# Flytta detta till if [[ ${TAB2[4]} != "$(eval_gettext 'None')" ]]; then
# För att skippa "Use hinting" kryssrutan
echo -n "gtk-xft-hinting = " >> $rc_file
[[ ${TAB2[3]} == TRUE ]] && echo 1 >> $rc_file || echo 0 >> $rc_file
# End Flytta
if [[ ${TAB2[4]} != "$(eval_gettext 'None')" ]]; then
case ${TAB2[4]} in
"Slight") echo 'gtk-xft-hintstyle = "hintslight"' >> $rc_file ;;
"Medium") echo 'gtk-xft-hintstyle = "hintmedium"' >> $rc_file ;;
"Full") echo 'gtk-xft-hintstyle = "hintful"' >> $rc_file ;;
esac
fi
if [[ ${TAB2[5]} != "$(eval_gettext 'None')" ]]; then
case ${TAB2[5]} in
"RGB") echo 'gtk-xft-rgba = "rgb"' >> $rc_file ;;
"BGR") echo 'gtk-xft-rgba = "bgr"' >> $rc_file ;;
"VRGB") echo 'gtk-xft-rgba = "vrgb"' >> $rc_file ;;
"VBGR") echo 'gtk-xft-rgba = "vbgr"' >> $rc_file ;;
esac
fi
echo >> $rc_file
echo "gtk-color-scheme = \"fg_color:${TAB3[0]};bg_color:${TAB3[1]};text_color:${TAB3[2]};base_color:${TAB3[3]};selected_fg_color:${TAB3[4]};selected_bg_color:${TAB3[5]};tooltip_fg_color:${TAB3[6]};tooltip_bg_color:${TAB3[7]}\"" >> $rc_file
echo -e "\n# Custom settings\ninclude \"$rc_file.mine\"" >> $rc_file
fi
# cleanup
rm -f $res1 $res2 $res3
![]() |
![]() |