1. Yad Progress
Display progress indication dialog.
#!/bin/bash
for ((i=1; i<=100; i++)) {
echo $i
echo "# $((i))%"
sleep 0.5
} | yad --progress \
--text="Progress demo..." \
--width=300 \
--button=yad-cancel \
--auto-kill
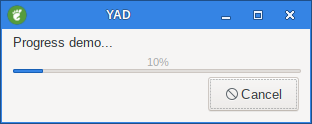
When the --progress
option is used, yad reads lines of progress data from stdin.
When the lines begin with the text after
is displayed in the progress barlabel.
Numeric values treats like a persents for progress bar.
1.1. --pulsate
Pulsate progress bar.
This option works only in single-bar mode.
#!/bin/bash
for ((i=1; i<=100; i++)) {
echo $i
echo "# $((i))%"
sleep 0.2
} | yad --progress \
--pulsate \
--text="Pulsate..." \
--width=300 \
--button=yad-cancel \
--auto-kill
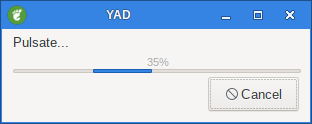
1.2. --auto-close
Dismiss the dialog when 100% has been reached.
1.3. --auto-kill
Kill parent process if cancel button is pressed.
1.4. --progress-text=TEXT
Set the label of progress bar to TEXT
.
This option works only in single-bar mode.
yad --progress --progress-text=Demo 20
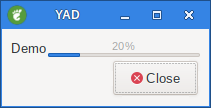
1.5. --rtl
Right-To-Left progress bar direction.
This option works only in single-bar mode.
#!/nin/bash
for ((i=1; i<=100; i++)) {
echo $i
echo "# $((i))%"
sleep 0.2
} | yad --progress \
--text="Progress demo..." \
--width=300 \
--button=yad-cancel \
--auto-kill --rtl
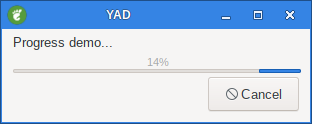
1.6. --vertical
Set vertical orientation of progress bars.
yad --progress --vertical 20
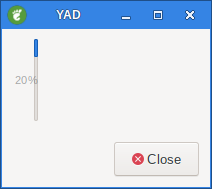
1.7. --enable-log=[TEXT]
Show log window.
#!/bin/sh
for ((i=1; i<=100; i++)) {
echo $i
echo "# Remaining $((100-i))% to finish the job"
sleep 0.2
} | yad --progress \
--enable-log="Test Log" \
--text="Testing..." \
--width=300 \
--button=yad-cancel \
--auto-kill
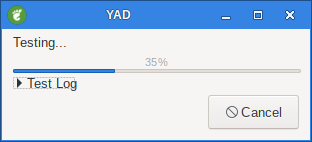
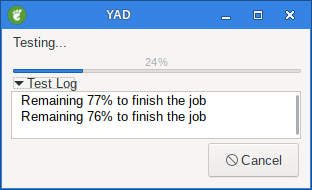
1.8. --log-expanded
Expand log window.
#!/bin/sh
for ((i=1; i<=100; i++)) {
echo $i
echo "# Remaining $((100-i))% to finish the job"
sleep 0.2
} | yad --progress \
--enable-log="Test Log" \
--text="Testing..." \
--width=300 \
--button=yad-cancel \
--log-expanded \
--auto-kill
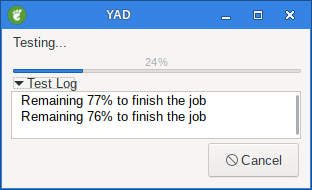
1.9. --log-height
--log-height
Height of log window.
#!/bin/sh
for ((i=1; i<=100; i++))
{
echo $i
echo "# Remaining $((100-i))% to finish the job"
sleep 0.2
} | yad --progress \
--log-height=100 \
--enable-log="Test Log" \
--log-expanded \
--text="Testing..." \
--width=300 \
--button=yad-cancel \
--auto-kill
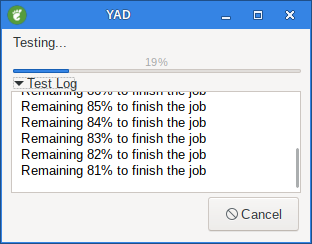
2. Yad Multi progress bars
Initial values for bars sets as an extra arguments.
Each lines with progress data passed to stdin must be started from N: where N is a number of progress bar.
In a single-bar mode N: is not needed.
yad --progress \
--width=250 \
--bar="Bar 1" 10 \
--bar="Bar 2" 20
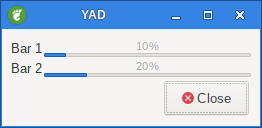
2.1. --bar=LABEL[:TYPE]
Add progress bar.
LABEL
is a text label for progress bar.
TYPE
is a progress bar type.
Types are: NORM
for normal progress bar, RTL
for inverted progress bar and PULSE
for pulsate progress bar.
If no bars specified, the progress dialog works in single-bar mode.
yad --progress \
--width=250 \
--bar="Bar 1:rtl" 10 \
--bar="Bar 2:rtl" 20
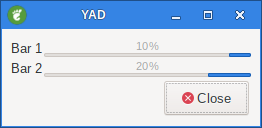
2.2. --watch-bar=NUMBER
Watch for specific bar for auto close
2.3. --auto-close
Dismiss the dialog when 100% of all bars has been reached
2.4. --auto-kill
Kill parent process if cancel button is pressed
3. Examples
3.1. xdf
Here is an excellent example of multiple progress bars. It will only show the drives that are currently mounted.
#!/bin/sh
eval exec yad \
--title="xdf" \
--image=drive-harddisk \
--text="Disk\ usage:" \
--buttons-layout=end \
--width=650 \
--progress \
$(df -hT $1 | tail -n +2 | \
awk '{printf "--bar=\"<b>%s</b> (%s - %s) [%s/%s]\" %s ", $7, $1, $2, $4, $3, $6}')
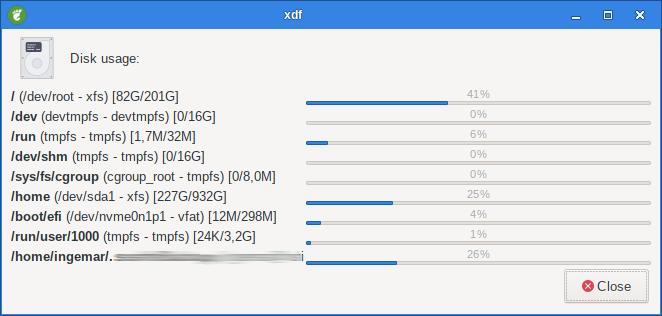